WordPress is one of the most secure content management systems (CMS) you can use to run a website. However, like any software, it has its vulnerabilities and security challenges. If you’re unaware of these issues or how to address them, even the best security measures might not be enough to protect your website.
The positive aspect is that securing WordPress sites is generally easier compared to other platforms because you can use different security plugins and functions.
In this article, we’ll explore the most common problems that WordPress site owners face and their solutions.
Most Common WordPress Vulnerabilities
1. SQL Injection
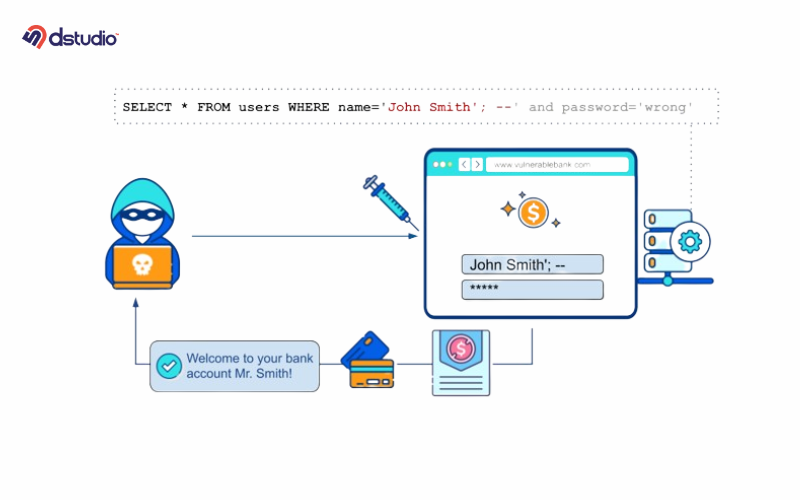
SQL injection occurs when a website fails to check or approve the information that users enter before using it in a database query. This problem can also happen if the input validation is done wrong or if WordPress functions are not used correctly. Because of this, harmful SQL code can be added, which creates major security risks.
Risk Level
SQL injection can have a really serious impact because it can damage the security and privacy of a website. It lets attackers get into sensitive information or insert harmful data into the database.
Example
Here are some examples of weak code where user input is used in SQL queries without enough security measures:
$variable = $_REQUEST['id'];
// Vulnerable, user input variable used directly in SQL query.
$wpdb->get_var("SELECT * FROM wp_users WHERE id = " . $variable);
// Vulnerable, esc_sql variables must be enclosed within quotes.
$wpdb->get_var("SELECT * FROM wp_users WHERE id = " . esc_sql($variable));
// Vulnerable, sanitize_text_field does not remove quotes or escape values.
$wpdb->get_var("SELECT * FROM wp_users WHERE id = " . sanitize_text_field($variable));
In each of these situations, user input is added straight into SQL queries, which puts the database at risk.
How to Fix SQL Injection Problems
To stop SQL injection attacks, always use $wpdb->prepare when making database queries that include user input. This method helps you safely use placeholders (like %s for strings and %d for numbers) instead of putting user input directly into the query.
Secure Code Example:
$variable = intval($_REQUEST['id']);
$wpdb->get_var($wpdb->prepare("SELECT * FROM wp_users WHERE id = %d", [$variable]));
2. Cross-Site Scripting (XSS)
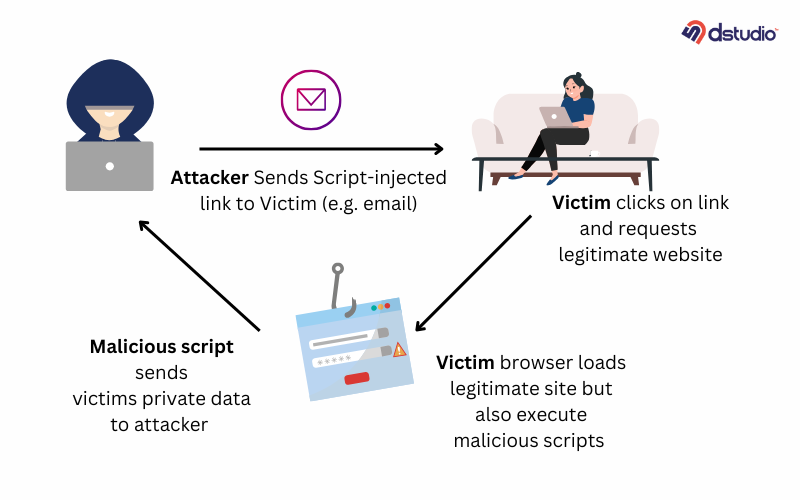
Cross-Site Scripting (XSS) happens when user-provided data is displayed on a webpage without being properly checked or secured. If input isn’t sanitized (cleaned up) or escaped (made safe for display), attackers can insert malicious scripts that run on the site.
This issue often arises when developers either skip these checks or use WordPress functions incorrectly. As a result, attackers can use XSS to harm the site by redirecting users, displaying fake content, or executing unauthorized code.
Risk Level
XSS is a critical vulnerability that can seriously impact a site’s security.
Example of Vulnerable Code:
Here’s an example of vulnerable code, where user input is directly displayed without proper escaping:
$identifier = get_option('my_identifier');
// Vulnerable, zero output escaping.
echo '<input type="text" name="my_identifier" value="' . $identifier . '">';
// Vulnerable, sanitize_text_field does not escape quotes.
echo '<input type="text" name="my_identifier" value="' . sanitize_text_field($identifier) . '">';
In each case, the input isn’t escaped, leaving the site open to XSS attacks.
How to Fix Cross-Site Scripting Vulnerabilities
WordPress offers several escape functions to secure user input before displaying it. The function you use depends on the context (e.g., HTML attributes, text content). When displaying user-provided values inside HTML attributes, use esc_attr()
to prevent XSS.
Secure Code Example:
$identifier = get_option('my_identifier');
echo '<input type="text" name="my_identifier" value="' . esc_attr($identifier) . '">';
Shortcode Vulnerabilities
XSS vulnerabilities can also appear in plugins that allow user-generated shortcodes. Always escape any user-provided data within shortcodes.
Example of Securing Shortcode Output:
extract( shortcode_atts( array(
'style' => ''
), $params ) );
// Vulnerable:
$html = '<div style="' . $style . '" class="my class">';
// Should be:
$html = '<div style="' . esc_attr($style) . '" class="my class">';
// Some other code here to generate the HTML...
return $html;
3. Broken Access Control
Broken access control vulnerabilities occur when a website does not properly check a user’s authorization or authentication. This often happens with actions registered under specific hooks in WordPress, such as wp_ajax_*
, admin_action_*
, admin_post_*
, admin_init
, and register_rest_route
.
By default, these hooks do not verify if a user has the right permissions. For example, in register_rest_route
, this issue can arise when the permission callback function always returns true, allowing unauthorized access.
Risk Level
The severity of broken access control is very high. Many vulnerabilities stem from a lack of authorization checks, often leading to unauthorized changes in plugin settings.
Example of a Vulnerable Code
Here’s an example that registers a WordPress AJAX action. This action is registered under wp_ajax
, which means it requires at least subscriber-level permissions:
// Example without nonce token check
add_action('wp_ajax_update_settings', function(){
update_option("my_settings", $_POST);
});
How to Fix Broken Access Control Vulnerabilities
To prevent broken access control, WordPress offers functions like current_user_can and user_can to verify a user’s permissions. These should be implemented to ensure that only authorized users can perform actions that require higher privileges.
Additionally, it’s crucial to check nonce tokens to protect against Cross-Site Request Forgery (CSRF) attacks.
Secure Code Example:
add_action('wp_ajax_update_settings', function() {
// Check user permissions and validate the nonce token
if (!current_user_can('manage_options') || !wp_verify_nonce('action', 'action')) {
exit;
}
// Update settings securely
update_option("my_settings", [
'setting1' => esc_html($_POST['setting1'])
]);
});
In this secure example, we check if the user has the necessary permissions and if the nonce token is valid before allowing any updates to the settings.
4. Cross-Site Request Forgery (CSRF)
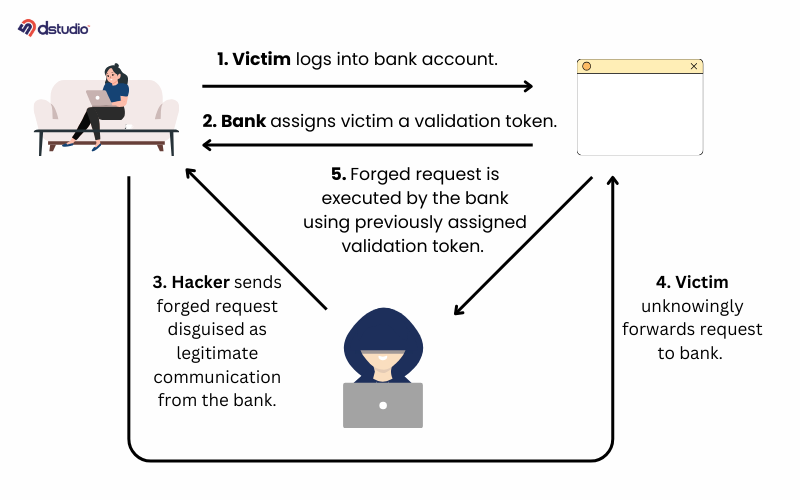
Cross-Site Request Forgery (CSRF) occurs when a nonce token is not validated during an authorized action.
For instance, if you have a WordPress AJAX action that checks user authorization but ignores nonce validation, an attacker could trick a higher-privileged user into executing an action with harmful values.
This could happen by making the user visit a malicious site that contains the CSRF payload or through an existing cross-site scripting (XSS) vulnerability on the same site.
Risk Level
The severity of CSRF varies. It is not commonly exploited because it typically relies on tricking a higher-privileged user, which often requires social engineering tactics.
Example of a Vulnerable Code
Here’s an example of an AJAX action that checks if a user has permission but does not validate the nonce token:
add_action('wp_ajax_update_settings', function() {
if (!current_user_can('manage_options')) {
exit;
}
update_option("my_settings", [
'setting1' => esc_html($_POST['setting1'])
]);
});
In this case, even though the user’s authorization is checked, the lack of nonce validation leaves it vulnerable to CSRF attacks.
How to Prevent CSRF Vulnerabilities
WordPress gives you some tools to check if a nonce token is valid. First, you need to make sure the nonce token is given to the user. You can create a nonce token for the front end by using the wp_create_nonce function.
Once you have the nonce value, you can check it using the wp_verify_nonce function in an if statement. Alternatively, you can use the check_admin_referer function without an if statement, which will automatically stop the script if it can’t validate the nonce token.
Secure Code Example:
Here’s how you can properly implement nonce validation in your AJAX action:
add_action('wp_ajax_update_settings', function() {
if (!current_user_can('manage_options') || !wp_verify_nonce('action', 'action')) {
exit;
}
update_option("my_settings", [
'setting1' => esc_html($_POST['setting1'])
]);
});
This code ensures that both the user has the required permissions and that the nonce token is validated.
Mistake to Avoid
It’s crucial to implement the nonce check correctly. Many developers make mistakes that can be taken advantage of.
For example, the following code still leaves the application vulnerable because if the action
POST parameter is missing, the nonce validation will not be executed:
add_action('wp_ajax_update_settings', function() {
if (!current_user_can('manage_options')) {
exit;
}
// Vulnerable implementation
if (isset($_POST['action']) && !wp_verify_nonce('action', 'action')) {
exit;
}
update_option("my_settings", [
'setting1' => esc_html($_POST['setting1'])
]);
});
In this case, if the action
parameter is not provided in the CSRF payload, the nonce token validation will be skipped, allowing potential CSRF attacks to occur. Always ensure that your nonce validation is robust and thoroughly tested.
5. Server-Side Request Forgery (SSRF)
Server-side Request Forgery happens when a user can enter a URL in an HTTP request, which lets them fool the server into making requests to any URL they want. This can be very risky, especially if there are local services running that aren’t open to the public, because attackers could get into those services.
Risk Level
The seriousness of SSRF vulnerabilities depends on what services are being used in the private environment. In a normal WordPress setup, the impact is usually low.
But if the results of an HTTP request are shown to the user, the risk goes up. This is because blind SSRF attacks—where the attacker can’t see the response—are usually harder to carry out successfully.
Example of Vulnerable Code:
wp_remote_get($_GET['url']);
How to Prevent SSRF
To prevent SSRF attacks, always validate the URL before using it in an HTTP request. Consider using the wp_safe_remote_get function instead of wp_remote_get
, or use safer alternatives like file_get_contents
or cURL with proper validations.
6. Directory Traversal
Directory traversal, also called path traversal or arbitrary file read, happens when someone tries to access files on a server’s storage and can view their contents. It’s important to note that this is different from Local File Inclusion (LFI), which usually means running a file on the server.
Risk Level
The severity of directory traversal vulnerabilities can range from medium to very high, depending on the information stored on the server and the site’s configuration.
Example of Vulnerable Code:
echo file_get_contents($_GET['file']);
How to Prevent Directory Traversal
To mitigate directory traversal vulnerabilities, validate input parameters before using them in any file-reading function.
You can compare the input against a list of acceptable values, ensure it contains only letters using ctype_alpha, or strip out slashes and dots. The specific fix will depend on how the file-reading function is implemented.
7. Local File Inclusion (LFI)
Local File Inclusion happens when a website allows a file to be included and run on the server using PHP. This can be very dangerous, especially if users can upload any type of file, no matter the file extension.
For example, if a file named image.png is included using PHP functions like include or require, any PHP code in that file will also run.
Risk Level
The risk level can range from medium to very high. It depends on what files are on the website and whether users can upload their own files.
Example
include $_GET['test'];
require_once './some/folder/' . $_GET['test'];
How to Prevent LFI
You should check the input value before using it in any function that includes a file. You can compare it to a list of safe values, make sure it only has letters using a function like ctype_alpha, or remove all slashes and dots. Remember, how you fix this depends on how you’re using the function.
8. Remote File Inclusion (RFI)
Remote File Inclusion happens when a hacker tricks the server into loading a file from the internet and running it. This usually requires a setting in PHP called allow_url_include to be turned on.
Risk Level
The risk level is very high. This can lead to losing control over the website and exposing sensitive information.
Example
include $_GET['test'];
How to Prevent RFI
You should never allow loading files from remote URLs like this. If you really need to, make sure the user-provided value is on a list of allowed values or follows a strict pattern.
9. Remote Code Execution (RCE)
Remote Code Execution happens when user input is used in a PHP function that runs shell commands. Some functions that can do this include shell_exec, exec, popen, system, passthru, and proc_open.
Severity
The risk level is very high. Although it also depends on how the hosting environment and PHP are set up, this can still cause serious problems. Under certain conditions, someone could upload harmful scripts or gain unauthorized access.
Example
shell_exec('imgoptimize ' . $_GET['cmd']);
How to Prevent RCE
You must check the user input to ensure it only has allowed values. If you need to escape arguments used in a command, consider using escapeshellarg. Just remember that how you fix this will depend on how the user input is used in the command.
10. CSV Injection
CSV Injection happens when data provided by users is directly added to a CSV file that gets exported. If someone opens this CSV file in a program like Windows Excel, the harmful data could include a formula that runs a command instead of just showing text.
Risk Level
The severity of this issue is low to medium. It requires that the exported CSV file be opened in a program that allows such actions to occur. To exploit this vulnerability, it involves multiple steps and might require convincing a user with higher privileges to export or download the CSV file and then open it.
How to Prevent CSV Injection
Fixing this issue is pretty simple. You need to protect certain characters related to functions by adding a quote before them. Below is an example of how to do this. You take the CSV row (which is a list of values for one row, usually passed to a function called fputcsv) and run it through this function to escape the right characters.
function get_encoded_row( $row ) {
$result = [];
foreach ( $row as $key => $value ) {
$encoded_value = $value;
if ( in_array( substr( (string) $value, 0, 1 ), [ '=', '-', '+', '@', "\t", "\r" ], true ) ) {
$encoded_value = "'" . $value;
}
$result[ $key ] = $encoded_value;
}
return $result;
}
11. Data Exposure
Data Exposure vulnerabilities happen when users with lower privileges can trigger actions that reveal sensitive information about the website or its users. We often see this issue in plugins that show WooCommerce order details or complete addresses.
Risk Level
This issue is also low to medium in severity. It mainly affects the confidentiality of the website.
How to Prevent Data Exposure
This vulnerability usually exists because of a lack of proper authorization or authentication checks. To fix this, the method that lets someone access information needs to include the right checks to stop data from leaking.
WordPress has several functions that check user authorization. For example, current_user_can and user_can.
12. Insecure Direct Object Reference (IDOR)
Insecure Direct Object Reference vulnerabilities happen when a certain action or endpoint does not properly check if the user has the right permissions to access the requested resource.
For instance, if there’s a page that shows orders based on an order ID in the URL using the ?order_id
parameter, lower-privileged users shouldn’t be able to change that number to view other customers’ orders.
Risk Level
Like the other vulnerabilities, this one is also low to medium in severity. It mainly impacts the confidentiality of the website.
How to Prevent Insecure Direct Object Reference
To fix this, the user ID linked to the resource should match with the currently logged-in user. For example, if there is an order with user_id 5
, then someone logged in as user_id 6
should not be able to see that order.
13. Open Redirect
An Open Redirect happens when a website sends a user to a different page based on a URL that the user provides, but it doesn’t check if that URL is safe. When redirecting based on a URL, it’s important to ensure that the URL doesn’t lead to a harmful website.
Risk Level
Low to medium. This issue is not often used by attackers and usually requires tricking users to work.
Example:
header('Location: ' . $_GET['url']);
// or
wp_redirect($_GET['url']);
How to Prevent Open Redirect
To safely redirect users, use the wp_safe_redirect
function. If the redirect URL is different from your site, use the allowed_redirect_hosts filter to add safe websites.
14. Privilege Escalation
Privilege Escalation happens when a user with low permissions or no account can take actions that give them higher privileges. This often means that someone who shouldn’t have access can log in as an administrator.
Risk Level
Very high. If a user can log in as an administrator, they can control the entire website, which can harm its security and reliability.
Example:
This problem often appears in plugins that allow logging in through social media. If these plugins don’t check the login properly, users can bypass the login process and access any account. This can happen because of functions like wp_set_auth_cookie or wp_set_current_user.
How to Prevent Privilege Escalation
Since each situation can be different, there isn’t one simple fix. It’s important to properly check if someone is authenticated before using WordPress functions that allow them to log in as another user.
15. Race Condition
Race Condition vulnerabilities happen in functions where users should only be able to do something once, like voting or rating. If a user sends many requests at the same time, the system might not notice that the action has already been completed, allowing it to happen multiple times.
Risk Level
Low to medium. This type of vulnerability is hard to fix with firewalls, but it’s rarely exploited.
How to Prevent Race Condition
For race conditions in SQL functions, you can use transaction locking, but that might be tricky in WordPress. Another option is to use mutex locks that depend on the filesystem. You can find an example of this implementation online.
16. Arbitrary File Upload
Arbitrary File Upload happens when a user uploads a file and the system doesn’t properly check the file type. This can let someone upload a harmful .php file, which could take over the entire website.
Risk Level
Very high. This can seriously affect the website’s security and availability.
Example:
move_uploaded_file($_FILES['file']['tmp_name'], '/wp-content/uploads/' . $_FILES['file']['name']);
How to Prevent Arbitrary File Upload
WordPress has its own way to upload files, so you should never use basic PHP functions for this. Instead, use the wp_handle_upload function, which checks the file type and extension automatically.
17. Arbitrary File Download
Arbitrary File Download occurs when a user can download any file from the server by giving a specific name. For example, if there’s an export button that uses a URL to download files, someone could change that URL to download any file they want.
Risk Level
Medium to very high. This depends on what kind of information is on the website. For example, if a sensitive file like wp-config.php is accessible, someone could gain important database connection details.
Example:
$file = $_GET['filename'];
header('Content-Description: File Transfer');
header('Content-Type: application/octet-stream');
header('Content-Disposition: attachment; filename="'.basename($file).'"');
header('Expires: 0');
header('Cache-Control: must-revalidate');
header('Pragma: public');
header('Content-Length: ' . filesize($file));
readfile($file);
exit;
How to Prevent Arbitrary File Download
Always check the input before using it to read a file. You can compare it to a list of allowed values, make sure it only has letters using `ctype_alpha`, or remove any slashes and dots. The exact fix depends on how the function is set up.
18. Arbitrary File Deletion
Arbitrary File Deletion vulnerabilities happen when a user can give a value that is used with PHP’s unlink function, which deletes files. If someone can change the file path, they could delete any file they want.
Risk Level
Medium to very high. This depends on how the file deletion is done. If user input is directly used in the unlink function, it becomes a very serious problem.
Example:
unlink('/wp-content/uploads/' . $_GET['file']);
How to Prevent Arbitrary File Deletion:
Always validate the input before using it to delete a file. You can compare it to a list of allowed values, check that it only contains letters with ctype_alpha, or remove slashes and dots. You might also want to use the wp_delete_file_from_directory` function to improve security.